일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | ||||||
2 | 3 | 4 | 5 | 6 | 7 | 8 |
9 | 10 | 11 | 12 | 13 | 14 | 15 |
16 | 17 | 18 | 19 | 20 | 21 | 22 |
23 | 24 | 25 | 26 | 27 | 28 |
Tags
- dart
- 쿠키런킹덤공략
- 쿠킹덤공략
- Eclipse
- 크리스마스
- Unity
- 쿠킹덤
- 웹개발
- programmers
- 딥러닝
- 자바
- 쿠키런킹덤
- 개발자
- MySQL
- SQL
- 자바스크립트
- 오블완
- 쿠키런킹덤크리스마스
- 유니티
- oracle
- 홀리데이익스프레스
- Spring
- 노마드코더
- JavaScript
- 이클립스
- Java
- 프로그래머스
- 티스토리챌린지
- edwith
- HTML
Archives
- Today
- Total
Dev study and notes
플러터 Flutter로 웹툰 앱 만들기 #5 POMODORO APP 새로운 앱 생성 - 타이머 본문
Building & Learning/Flutter로 웹툰앱 만들기(2023)
플러터 Flutter로 웹툰 앱 만들기 #5 POMODORO APP 새로운 앱 생성 - 타이머
devlunch4 2025. 1. 28. 06:10반응형
이번시간엔 간단한 앱으로 타이머를 만드는 강좌입니다.
#5 POMODORO APP
#5.0 User Interface (13:35)
main.dart 수정(소스)
import 'package:flutter/material.dart';
import 'package:toonflix/screens/home_screen.dart';
void main() {
runApp(const App());
}
class App extends StatelessWidget {
const App({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData(
colorScheme: ColorScheme.fromSwatch(
backgroundColor: const Color(0xFFE7626C),
),
textTheme: const TextTheme(
displayLarge: TextStyle(
color: Color(0xFF232B55),
),
),
cardColor: const Color(0xFFF4EDDB),
),
home: const HomeScreen(),
);
}
}
home_screen.dart 생성(소스)
import 'package:flutter/material.dart';
class HomeScreen extends StatefulWidget {
const HomeScreen({super.key});
@override
State<HomeScreen> createState() => _HomeScreenState();
}
class _HomeScreenState extends State<HomeScreen> {
@override
Widget build(BuildContext context) {
return Scaffold(
body: Column(
children: [
Flexible(
flex: 1,
child: Container(
decoration: const BoxDecoration(
color: Colors.red,
),
),
),
Flexible(
flex: 2,
child: Container(
decoration: const BoxDecoration(
color: Colors.green,
),
),
),
Flexible(
flex: 1,
child: Container(
decoration: const BoxDecoration(
color: Colors.blue,
),
),
),
],
),
);
}
}
flex 1 : 2 : 1 비율로 화면이 보여지게 됩니다.
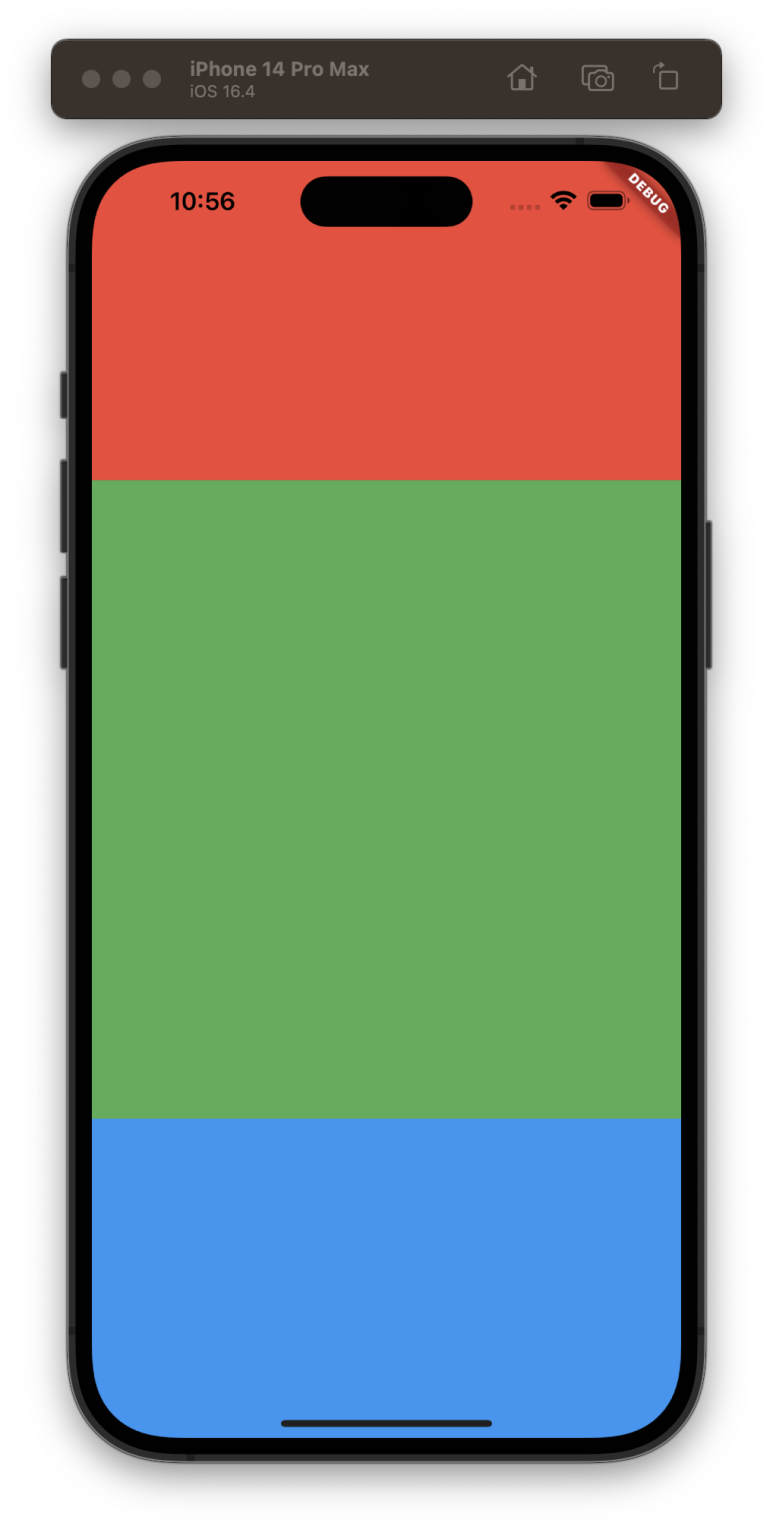
중간중간 소스를 추가/수정하면
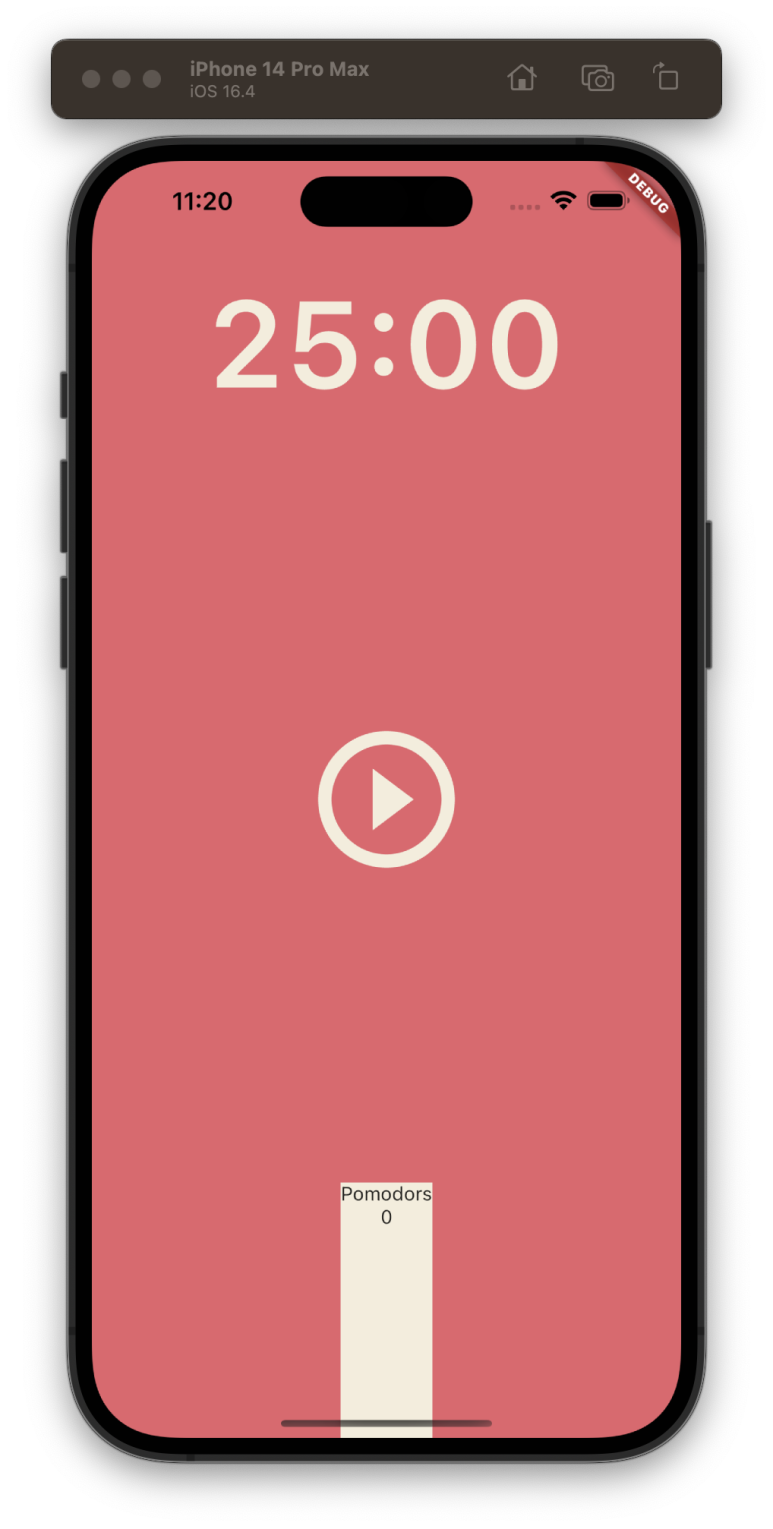
home_screen.dart (소스)
import 'package:flutter/material.dart';
class HomeScreen extends StatefulWidget {
const HomeScreen({super.key});
@override
State<HomeScreen> createState() => _HomeScreenState();
}
class _HomeScreenState extends State<HomeScreen> {
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: Theme.of(context).colorScheme.background,
body: Column(
children: [
Flexible(
flex: 1,
child: Container(
alignment: Alignment.bottomCenter,
child: Text(
"25:00",
style: TextStyle(
color: Theme.of(context).cardColor,
fontSize: 89,
fontWeight: FontWeight.w600,
),
),
),
),
Flexible(
flex: 3,
child: Center(
child: IconButton(
iconSize: 120,
color: Theme.of(context).cardColor,
onPressed: () {},
icon: const Icon(Icons.play_circle_outline),
),
),
),
Flexible(
flex: 1,
child: Row(
children: [
Expanded(
child: Container(
decoration: BoxDecoration(
color: Theme.of(context).cardColor,
),
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(
"Pomodors",
style: TextStyle(
fontSize: 20,
fontWeight: FontWeight.w600,
color:
Theme.of(context).textTheme.displayLarge!.color,
),
),
Text(
"0",
style: TextStyle(
fontSize: 58,
fontWeight: FontWeight.w600,
color:
Theme.of(context).textTheme.displayLarge!.color,
),
),
],
),
),
),
],
),
),
],
),
);
}
}
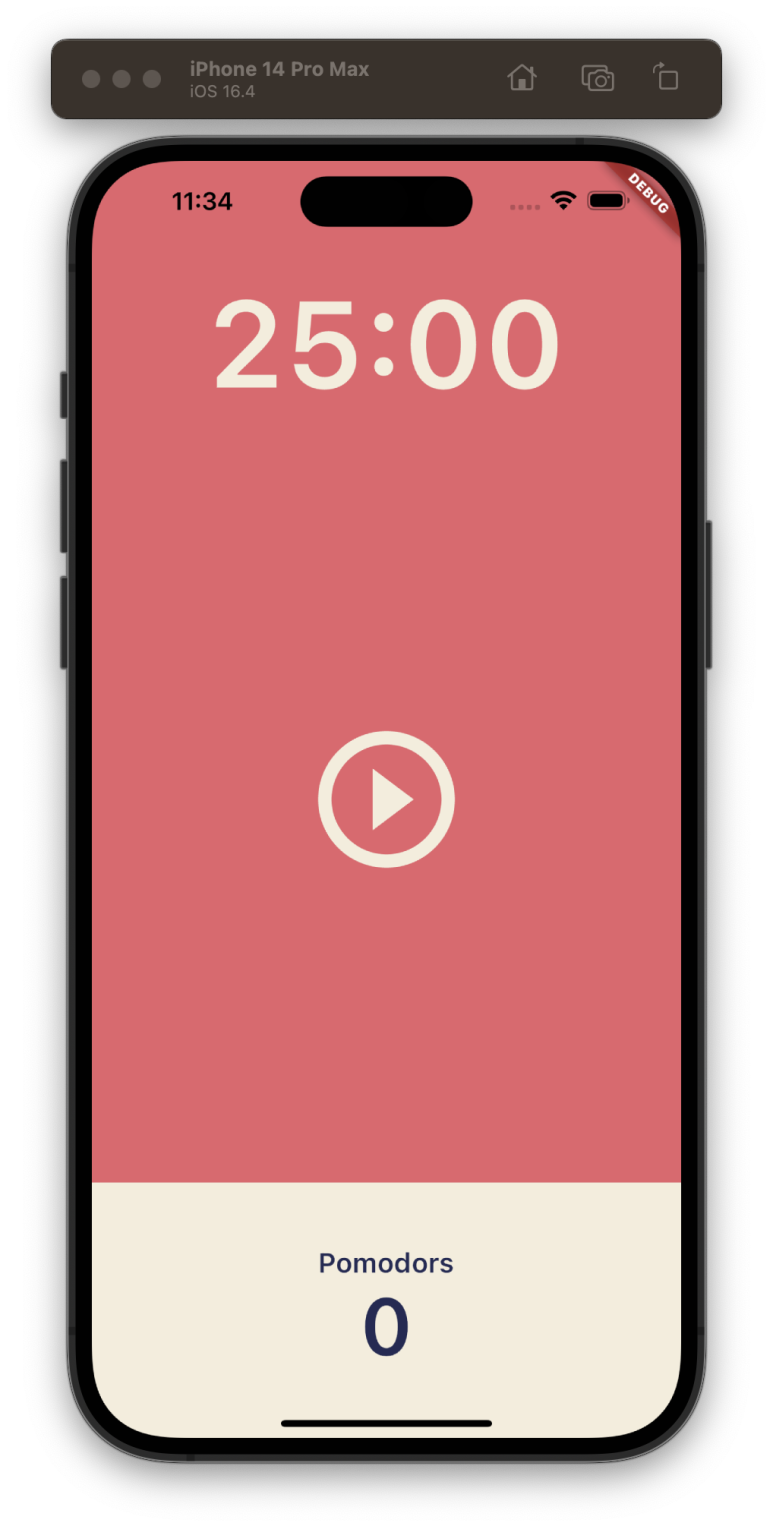
#5.1 Timer (05:48)
강의에 맞게 코딩합니다.
결과 : 타이머 버튼과 시간 감소 생성 완료
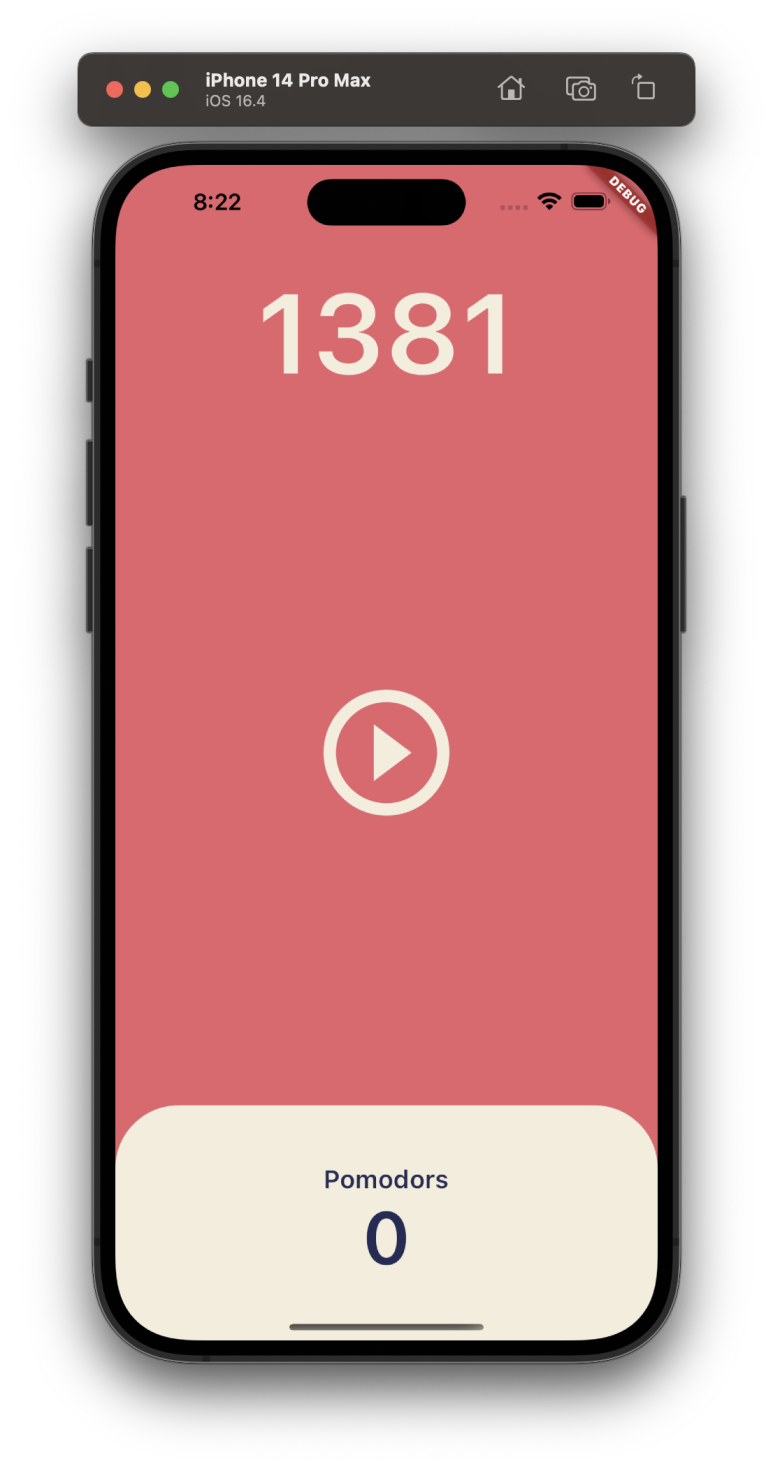
#5.2 Pause Play (04:26)
타이머를 시작/일시정지 합니다.
소스코드(아래)
import 'dart:async';
import 'package:flutter/material.dart';
class HomeScreen extends StatefulWidget {
const HomeScreen({super.key});
@override
State<HomeScreen> createState() => _HomeScreenState();
}
class _HomeScreenState extends State<HomeScreen> {
int totalSeconds = 1500;
bool isRunning = false;
late Timer timer;
void onTick(Timer timer) {
setState(() {
totalSeconds = totalSeconds - 1;
});
}
void onStartPressed() {
timer = Timer.periodic(
const Duration(seconds: 1),
onTick,
);
setState(() {
isRunning = true;
});
}
void onPausePressed() {
timer.cancel();
setState(() {
isRunning = false;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: Theme.of(context).colorScheme.background,
body: Column(
children: [
Flexible(
flex: 1,
child: Container(
alignment: Alignment.bottomCenter,
child: Text(
"$totalSeconds",
style: TextStyle(
color: Theme.of(context).cardColor,
fontSize: 89,
fontWeight: FontWeight.w600,
),
),
),
),
Flexible(
flex: 3,
child: Center(
child: IconButton(
iconSize: 120,
color: Theme.of(context).cardColor,
onPressed: isRunning ? onPausePressed : onStartPressed,
icon: Icon(isRunning
? Icons.pause_circle_outline
: Icons.play_circle_outline),
),
),
),
Flexible(
flex: 1,
child: Row(
children: [
Expanded(
child: Container(
decoration: BoxDecoration(
color: Theme.of(context).cardColor,
borderRadius: BorderRadius.circular(50),
),
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(
"Pomodors",
style: TextStyle(
fontSize: 20,
fontWeight: FontWeight.w600,
color:
Theme.of(context).textTheme.displayLarge!.color,
),
),
Text(
"0",
style: TextStyle(
fontSize: 58,
fontWeight: FontWeight.w600,
color:
Theme.of(context).textTheme.displayLarge!.color,
),
),
],
),
),
),
],
),
),
],
),
);
}
}
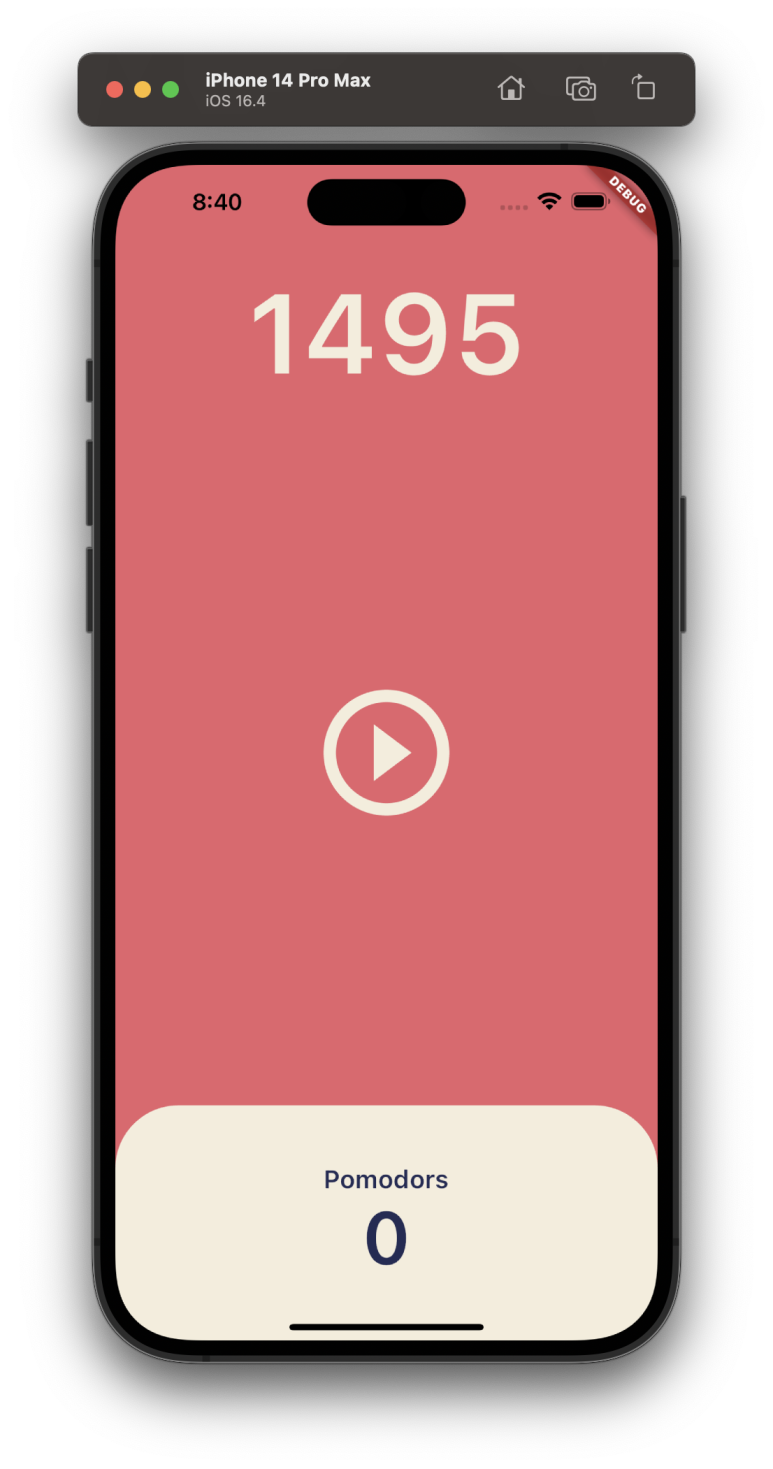
#5.3 Date Format (10:27)
초시간을 분시간으로 변경하였습니다.
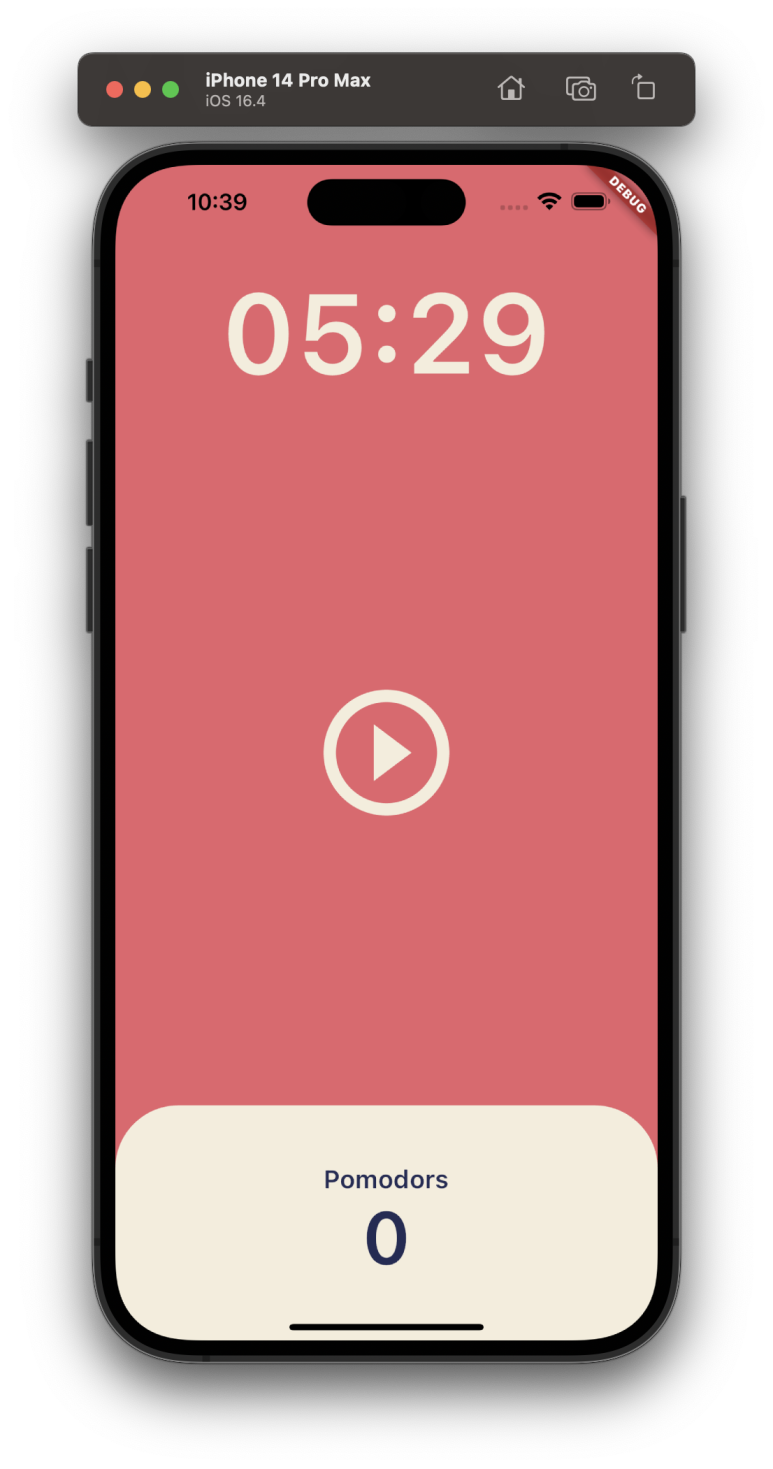
#5.4 Code Challenge (01:59)
reset 버튼 추가
소스코드
home_screen.dart
import 'dart:async';
import 'package:flutter/material.dart';
class HomeScreen extends StatefulWidget {
const HomeScreen({super.key});
@override
State<HomeScreen> createState() => _HomeScreenState();
}
class _HomeScreenState extends State<HomeScreen> {
static const twentyFiveMinute = 1500;
int totalSeconds = twentyFiveMinute;
bool isRunning = false;
late Timer timer;
int totalPomodoros = 0;
void onTick(Timer timer) {
if (totalSeconds == 0) {
setState(() {
totalPomodoros = totalPomodoros + 1;
isRunning = false;
totalSeconds = twentyFiveMinute;
});
timer.cancel();
} else {
setState(() {
totalSeconds = totalSeconds - 1;
});
}
}
void onStartPressed() {
timer = Timer.periodic(
const Duration(seconds: 1),
onTick,
);
setState(() {
isRunning = true;
});
}
void onPausePressed() {
timer.cancel();
setState(() {
isRunning = false;
});
}
void onResetPressed() {
timer.cancel();
setState(() {
totalSeconds = twentyFiveMinute;
isRunning = false;
});
}
String format(int seconds) {
var duration = Duration(seconds: seconds);
//print(duration.toString().split(".").first.substring(2, 7));
return duration.toString().split(".").first.substring(2, 7);
}
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: Theme.of(context).colorScheme.background,
body: Column(
children: [
Flexible(
flex: 1,
child: Container(
alignment: Alignment.bottomCenter,
child: Text(
format(totalSeconds),
style: TextStyle(
color: Theme.of(context).cardColor,
fontSize: 89,
fontWeight: FontWeight.w600,
),
),
),
),
Flexible(
flex: 2,
child: Center(
child: IconButton(
iconSize: 120,
color: Theme.of(context).cardColor,
onPressed: isRunning ? onPausePressed : onStartPressed,
icon: Icon(isRunning
? Icons.pause_circle_outline
: Icons.play_circle_outline),
),
),
),
Flexible(
flex: 1,
child: Center(
child: IconButton(
iconSize: 120,
color: Theme.of(context).cardColor,
onPressed: isRunning ? onResetPressed : onResetPressed,
icon: const Icon(Icons.restore_outlined),
),
),
),
Flexible(
flex: 1,
child: Row(
children: [
Expanded(
child: Container(
decoration: BoxDecoration(
color: Theme.of(context).cardColor,
borderRadius: BorderRadius.circular(50),
),
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(
"Pomodors",
style: TextStyle(
fontSize: 20,
fontWeight: FontWeight.w600,
color:
Theme.of(context).textTheme.displayLarge!.color,
),
),
Text(
"$totalPomodoros",
style: TextStyle(
fontSize: 58,
fontWeight: FontWeight.w600,
color:
Theme.of(context).textTheme.displayLarge!.color,
),
),
],
),
),
),
],
),
),
],
),
);
}
}
끝!
반응형
'Building & Learning > Flutter로 웹툰앱 만들기(2023)' 카테고리의 다른 글
플러터 Flutter로 웹툰 앱 만들기 #6 WEBTOON toonflix APP (0) | 2025.01.28 |
---|---|
플러터 Flutter로 웹툰 앱 만들기 #4 STATEFUL WIDGETS 새로운 앱 생성 - (0) | 2025.01.28 |
플러터 Flutter로 웹툰 앱 만들기 #3 UI CHALLENGE 플러터개발자도구 요소 스크롤 박스 (1) | 2025.01.28 |
플러터 Flutter로 웹툰 앱 만들기 #2 HELLO FLUTTER _ install in Mac MacOs 맥 플러터 설치 환경변수 설정 (0) | 2025.01.28 |
플러터 Flutter로 웹툰 앱 만들기 #1 소개 요약정리 플러터 독학 (0) | 2025.01.28 |
Comments